Power of Recursion: A Guide to Understanding and Leveraging Recursive Algorithms
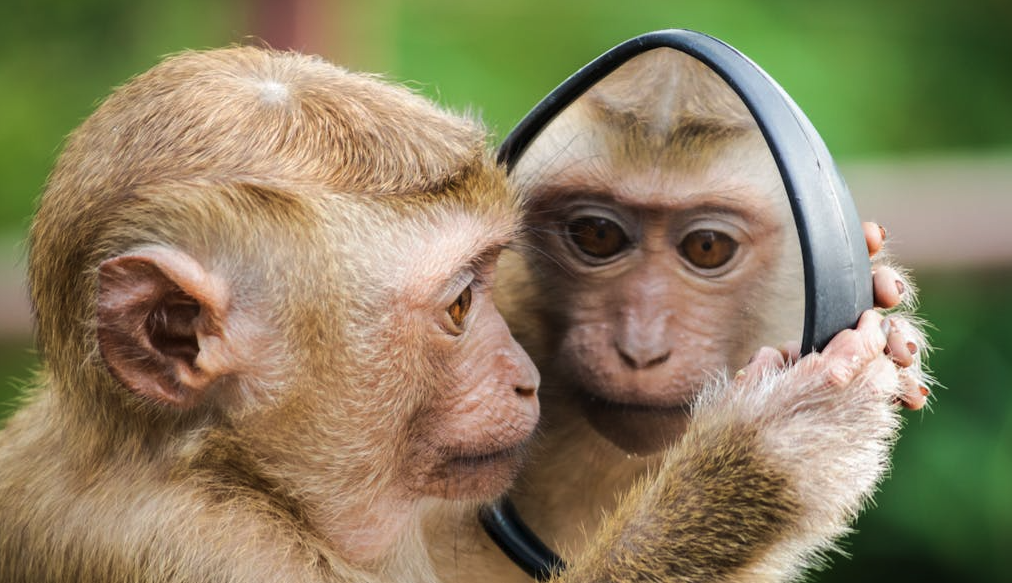
Date: 24/5/2024
Recursion is a fundamental concept in computer science and programming that empowers developers to solve complex problems by breaking them down into smaller, more manageable subproblems. While recursion may seem daunting at first, mastering this powerful technique opens up a world of possibilities for solving a wide range of problems efficiently and elegantly. In this article, we'll explore the concept of recursion, its uses, benefits, and common pitfalls, and provide practical examples to illustrate its application.
Let's explore a couple of practical examples to illustrate the use of recursion:
Factorial Calculation:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
Fibonacci Sequence:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(6)) # Output: 8
Understanding Recursion
At its core, recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. This process continues until a base case is reached, at which point the function returns a result. Recursion can be thought of as a way to break down a problem into simpler, more manageable subproblems, similar to how a Russian nesting doll contains smaller dolls within itself.
Common Use Cases for Recursion
Recursion is particularly well-suited for solving problems that exhibit recursive structure, such as tree traversal, pathfinding, and sorting algorithms. Some common use cases for recursion include:
Tree Traversal:
Recursively traverse tree data structures, such as binary search trees or linked lists, to perform operations like searching, insertion, deletion, or traversal.
Pathfinding Algorithms:
Solve maze problems or find the shortest path in a graph using recursive backtracking algorithms, such as depth-first search (DFS) or breadth-first search (BFS).
Sorting Algorithms:
Implement recursive sorting algorithms, such as merge sort or quicksort, which divide the input array into smaller subarrays, recursively sort each subarray, and then merge or combine the sorted subarrays.
Benefits of Recursion
Recursion offers several benefits, including:
Simplicity:
Recursive solutions often mirror the problem's natural recursive structure, making them intuitive and easy to understand.
Conciseness:
Recursive solutions are typically more concise and expressive than their iterative counterparts, reducing the amount of code needed to solve a problem.
Versatility:
Recursion can be applied to a wide range of problems and data structures, making it a versatile tool for solving complex problems.
Common Pitfalls and Challenges
Potential pitfalls and challenges :
Stack Overflow:
Recursion can lead to stack overflow errors if not implemented correctly or if the recursion depth exceeds the system's stack size.
Performance Overhead:
Recursive solutions may incur a performance overhead due to function call overhead and increased memory usage.
Difficulty Debugging:
Recursive code can be challenging to debug and reason about, especially when dealing with deep recursion or complex data structures.
Conclusion
Recursion is a powerful and versatile technique that empowers developers to solve complex problems efficiently and elegantly. By understanding the underlying principles of recursion, identifying suitable use cases, and mastering common recursion patterns, developers can leverage this fundamental concept to tackle a wide range of programming challenges with confidence and clarity. While recursion may seem intimidating at first, with practice and perseverance, it becomes a valuable tool in every programmer's toolkit.
Want to know more?
Seeking for professional advices?
Wilson Wong is an experienced professional developer.
If you have any questions about the topic or want to create a project.
Don't hesitate to achieve the goal together!
Hot topics
Let`s
start
a project
with us
Are you prepared for an exciting new project?
We are ready! Before we convene, we would appreciate it if you could provide us with some details. Kindly complete this form, or feel free to contact us directly via email if you prefer.