Exploring 3 Common Design Patterns (Three) - Factory Method Pattern
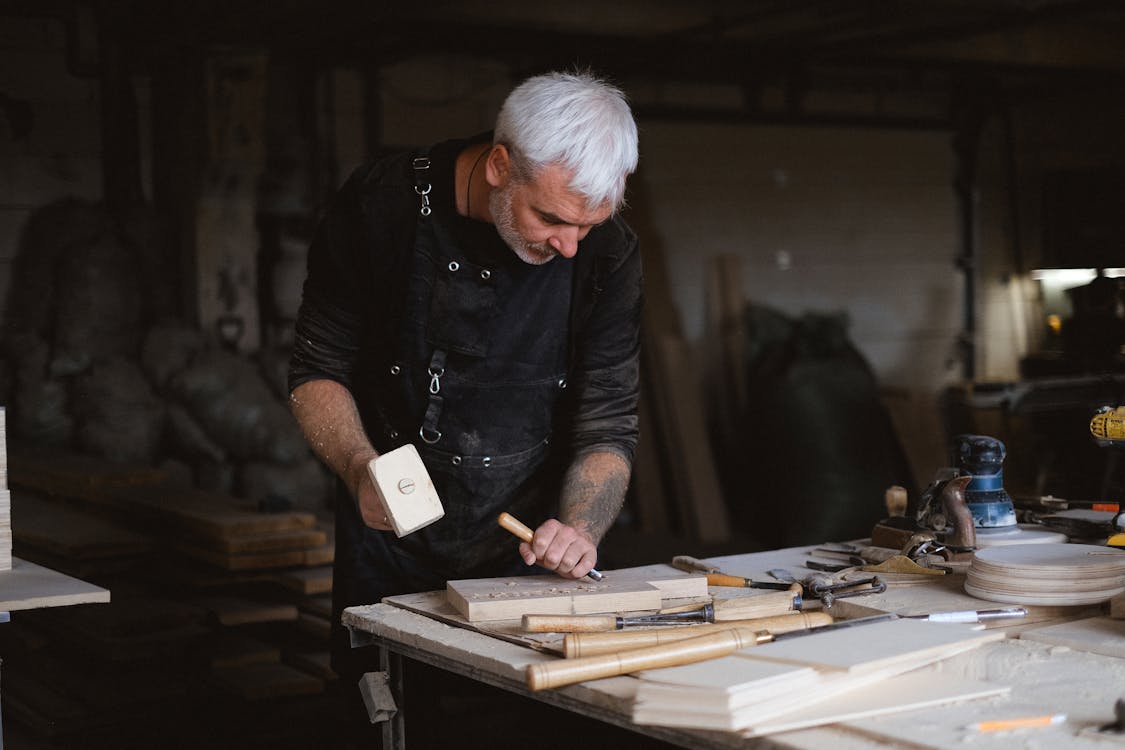
Date: 6/5/2024
Let's delve into the Factory Method Pattern this time. This pattern defines an interface for creating objects but delegates the responsibility of instantiation to its subclasses. This allows subclasses to determine which concrete class to instantiate based on specific requirements or conditions. But what exactly does this mean? Let's explore an example:
<!-- Product interface -->
class Animal {
speak() {
throw new Error("Method 'speak()' must be implemented.");
}
}
<!-- Concrete Product: Dog -->
class Dog extends Animal {
speak() {
return "Woof!";
}
}
<!-- Concrete Product: Cat -->
class Cat extends Animal {
speak() {
return "Meow!";
}
}
<!-- Creator interface -->
class AnimalFactory {
createAnimal() {
throw new Error("Method 'createAnimal()' must be implemented.");
}
}
<!-- Concrete Creator: Dog Factory -->
class DogFactory extends AnimalFactory {
createAnimal() {
return new Dog();
}
}
<!-- Concrete Creator: Cat Factory -->
class CatFactory extends AnimalFactory {
createAnimal() {
return new Cat();
}
}
<!-- Client code -->
<!-- Creating a Dog using DogFactory -->
const dogFactory = new DogFactory();
const dog = dogFactory.createAnimal();
console.log(dog.speak()); // Output: Woof!
<!-- Creating a Cat using CatFactory -->
const catFactory = new CatFactory();
const cat = catFactory.createAnimal();
console.log(cat.speak()); // Output: Meow!
The Factory Method Pattern typically comprises the following components:
Concrete Creator: Subclasses of the Creator implement the factory method to create instances of concrete products. Each Concrete Creator may offer a distinct implementation of the factory method, resulting in the creation of different types of objects.
Product: Objects created by the factory method. Products are typically defined by an interface or an abstract class, facilitating a common interface for client usage.
Concrete Product: Specific implementations of the Product interface or abstract class. Concrete Products are created by Concrete Creators through the factory method.
Distinguishing Between Creator and Product
The Creator defines the factory method and delegates the creation of products to its subclasses. Meanwhile, the Product represents the objects created by the factory method and defines their common interface or abstract class.
In Summary
The Factory Method Pattern is a potent design pattern offering a flexible and extensible solution for object creation in software systems. By delegating instantiation responsibilities to subclasses, the pattern encourages code reuse, maintainability, and scalability.
Want to know more?
Seeking for professional advices?
Wilson Wong is an experienced professional developer.
If you have any questions about the topic or want to create a project.
Don't hesitate to achieve the goal together!
Hot topics
Let`s
start
a project
with us
Are you prepared for an exciting new project?
We are ready! Before we convene, we would appreciate it if you could provide us with some details. Kindly complete this form, or feel free to contact us directly via email if you prefer.