Exploring 3 Common Design Patterns (Four) - Observer Pattern
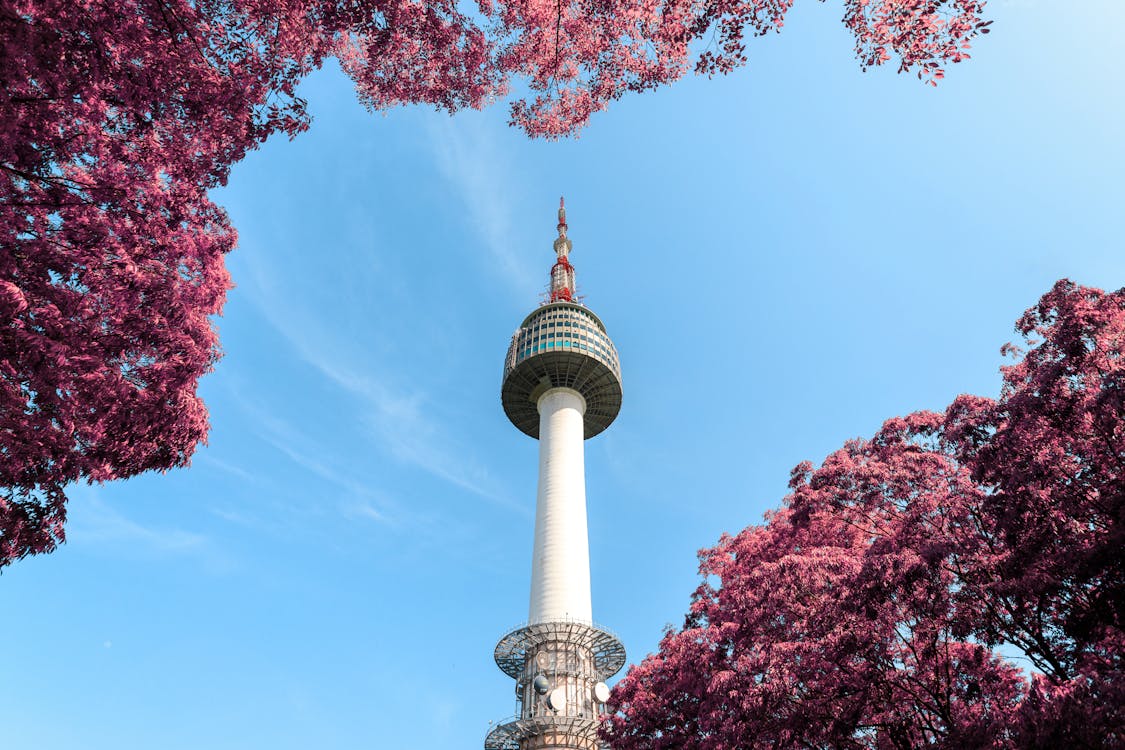
Date: 6/5/2024
The Observer Pattern is a cornerstone blueprint for crafting reactive and event-driven systems. It orchestrates a one-to-many relationship between objects, where a subject or publisher notifies its observers or subscribers of any state alterations, enabling automatic updates and synchronization across system components. This design fosters a reactive and loosely coupled architecture, enhancing system flexibility and responsiveness.
Illustrating the Concept:
Imagine a weather station serving as the subject, diligently monitoring weather conditions. Various displays, including digital screens, mobile apps, and notification services, act as observers keen on receiving weather updates. Upon detecting changes in temperature, humidity, or other metrics, the weather station promptly notifies all subscribed observers, prompting them to adjust their displays accordingly.
<!-- Observer interface -->
class Observer {
update() {
throw new Error("Method 'update()' must be implemented.");
}
}
<!-- Subject -->
class WeatherStation {
constructor() {
this.observers = [];
this.temperature = 0;
this.humidity = 0;
}
addObserver(observer) {
this.observers.push(observer);
}
removeObserver(observer) {
this.observers = this.observers.filter(obs => obs !== observer);
}
notifyObservers() {
this.observers.forEach(observer => observer.update(this.temperature, this.humidity));
}
setWeatherData(temperature, humidity) {
this.temperature = temperature;
this.humidity = humidity;
this.notifyObservers();
}
}
<!-- Concrete Observer: Digital Display -->
class DigitalDisplay extends Observer {
update(temperature, humidity) {
console.log(`Digital Display: Temperature is ${temperature}°C, Humidity is ${humidity}%`);
}
}
<!-- Concrete Observer: Mobile App -->
class MobileApp extends Observer {
update(temperature, humidity) {
console.log(`Mobile App: Weather Update - Temperature: ${temperature}°C, Humidity: ${humidity}%`);
}
}
<!-- Creating instances of subjects and observers -->
const weatherStation = new WeatherStation();
const digitalDisplay = new DigitalDisplay();
const mobileApp = new MobileApp();
<!-- Registering observers with the subject -->
weatherStation.addObserver(digitalDisplay);
weatherStation.addObserver(mobileApp);
<!-- Simulating weather data updates -->
weatherStation.setWeatherData(25, 60);
The Observer Pattern comprises several integral components:
This object is under observation. It manages a list of its observers and offers methods for registering, removing, and notifying observers of state changes.
This interface or abstract class is implemented by all observers. It defines a common method, such as update(), invoked by the subject to notify observers of state changes.
This concrete implementation of the subject interface maintains state and triggers notifications to its registered observers upon state alterations.
These concrete implementations of the observer interface register themselves with a subject to receive notifications and define behavior to execute upon receiving updates.
Conclusion
In summary, the Observer Pattern serves as a foundational element in crafting reactive software architectures, empowering developers to construct responsive and scalable systems. By establishing a flexible and decoupled architecture, this pattern facilitates seamless communication and collaboration between system components, fostering adaptability and maintainability. Whether utilized in user interfaces, event-driven systems, or real-time applications, the Observer Pattern remains a versatile and indispensable tool in the modern software engineer's arsenal.
Want to know more?
Seeking for professional advices?
Wilson Wong is an experienced professional developer.
If you have any questions about the topic or want to create a project.
Don't hesitate to achieve the goal together!
Hot topics
Let`s
start
a project
with us
Are you prepared for an exciting new project?
We are ready! Before we convene, we would appreciate it if you could provide us with some details. Kindly complete this form, or feel free to contact us directly via email if you prefer.